


What is WebSocket?
WebSocket is a protocol (RFC-6455) that enables full duplex communication between client and server. The core idea is that “client initiates a socket connection with server and then both the parties can exchange messages over single TCP connection”. This connection remains alive until manually closed or dropped out.
The overall difference between traditional HTTP and WebSocket can be explained as:
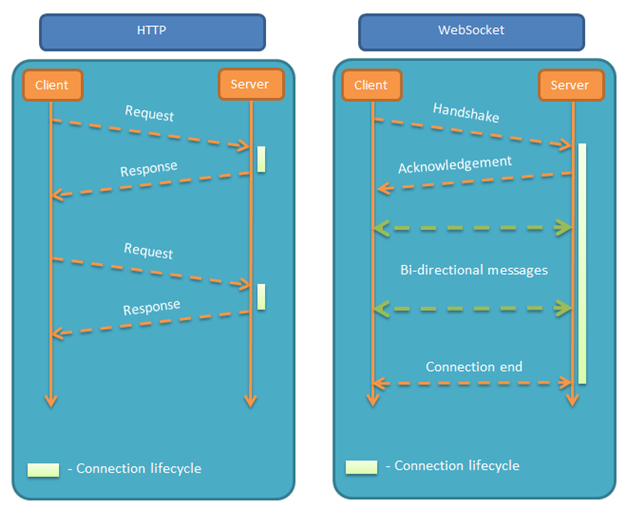
You can open a WebSocket connection by calling WebSocket constructor:
var connection = new WebSocket('ws://127.0.0.1:8080');
The “ws://” is a new URL schema for WebSocket connections. There is “wss://” which is also available for secure version.
How to communicate using WebSocket?
-
Setup server:
Use any one of the server side libraries and host a server to accept the client connections.
Server module will open a socket endpoint at a given TCP port.
For now let’s use Node JS based server implementation.
Install Node JS from: Nodejs.org
Create a directory and create the following server.js file in the same:
server.js
var port = 8081; //port to start server at var WebSocketNodeServer = require('ws').Server; var webSocketServer = new WebSocketNodeServer({port: port}); //start server //new conenction event handler webSocketServer.on('connection', function(wsInstance) { wsInstance.send('server: Welcome to websocket server.'); //send some welcome text //function to send server timestatmp value to client var sendServerTimeToClients = function(){ if(wsInstance.readyState == 1){ wsInstance.send('server: my clock is ' + (new Date()).toGMTString()); } }; //message event handler.. to handle message from client wsInstance.on('message', function(message) { wsInstance.send('server: ' +message); sendServerTimeToClients(); }); //send message to client every 2 seconds setInterval(function(){ sendServerTimeToClients(); }, 5000); }); console.log('WebSocketNodeServer started at: ' + port);
Then in the same directory, run the following commands using command prompt:
npm install ws node server.js
First command takes time to download and install ws node module. Once you run the second command, server starts a WebSocket endpoint at port 8081. Check comments in the code to know what each line does.
Server console:
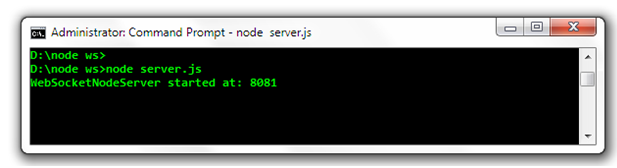
-
Setup client:
Initiate a connection by calling the connection constructor, this will return a connection object. The connection object provides events for handling various states of the connection.
These states are asynchronous in nature. It means connection can execute more than one state at a time.
So create a web page as below and host it on your local server.
Please provide valid ws socket server address with port number.
index.html
<!DOCTYPE html> <html> <head><title>WS client</title></head> <body> <script type="text/javascript"> //create connection object var connection = new WebSocket('ws://127.0.0.1:8081'); //function to send message to server function sendMessageToServer(){ var msg = document.getElementById('txtMsg').value; showMessage('me: '+ msg); connection.send(msg); } //just display given message on screen function showMessage(msgText){ var msg = document.createElement('li'); msg.innerHTML = msgText; var lst = document.getElementById('listMsgs'); lst.appendChild(msg); lst.scrollTop = lst.scrollHeight } //connection open event handler connection.onopen = function () { sendMessageToServer(); }; //message event handler .. handle message from server. connection.onmessage = function (e) { //console.log(e.data); showMessage(e.data); }; //connection close event handler .. handles connection close event connection.onclose = function(event) { console.log(event); }; </script> <!-- send a message to server when button is pressed --> <div><ul id="listMsgs" style="height:100px; overflow:auto;"></ul></div> <div> Enter message here: <input id="txtMsg" type="text" value="hello" /> <button onclick="sendMessageToServer()" > Send </button> </div> </body>
So what’s happening here?
A connection is getting established with server with this line of code and connection object is created.
var connection = new WebSocket('ws://127.0.0.1:8081');
The function sendMessageToServer sends a message over connection using connection.send() method.
The function connection.onopen is an event handler that gets fired once when a connection is opened first time.
The function connection.onmessage gets called back whenever there is some message from server.
Once connection is opened server sends a welcome message.
Whenever user presses the send message button, the message entered in input box is sent to server. The server simply echoes that message back to client. There is timer set on server which sends its own timestamp to client every five seconds. It means that the client can send message to server and server can also send message to client in an asynchronous manner.
Inspect the network tab of developer tools on the browser and you can see the WebSocket connection working live. You can see that incoming and outgoing messages are displayed in different colors here. This connection always remains in pending state to provide full duplex communication channel.
So this is all about simple implementation, now let me tell you what we have achieved here.
Once a client page is opened, a constant connection to server is established, using that connection we can send message to the server any time. At the same time the server can send me a message any time. Yes WebSocket is the technology behind the push notifications on our mobile phones.
So now we can develop stateful web applications!!
Where WebSocket is used?
- Instant messengers (IM)
- Push Notifications
- Online games
- Real time network apps
- Online games
- Live streams
- Real time dashboards